Java Code Examples for org.springframework.data.mongodb.core.convert.MappingMongoConverter
The following are top voted examples for showing how to useorg.springframework.data.mongodb.core.convert.MappingMongoConverter. These examples are extracted from open source projects. You can vote up the examples you like and your votes will be used in our system to product more good examples.
Project: spring-boot File: MongoDataAutoConfiguration.java View source code | 6 votes |
@Bean @ConditionalOnMissingBean(MongoConverter.class) public MappingMongoConverter mappingMongoConverter(MongoDbFactory factory, MongoMappingContext context, BeanFactory beanFactory) { DbRefResolver dbRefResolver = new DefaultDbRefResolver(factory); MappingMongoConverter mappingConverter = new MappingMongoConverter(dbRefResolver, context); try { mappingConverter .setCustomConversions(beanFactory.getBean(CustomConversions.class)); } catch (NoSuchBeanDefinitionException ex) { // Ignore } return mappingConverter; }
Project: kaa File: LogEventMongoDao.java View source code | 6 votes |
@SuppressWarnings("deprecation") public LogEventMongoDao(MongoDbConfig configuration) throws Exception { List<ServerAddress> seeds = new ArrayList<>(configuration.getMongoServers().size()); for (MongoDbServer server : configuration.getMongoServers()) { seeds.add(new ServerAddress(server.getHost(), server.getPort())); } List<MongoCredential> credentials = new ArrayList<>(); if (configuration.getMongoCredentials() != null) { for (MongoDBCredential credential : configuration.getMongoCredentials()) { credentials.add(MongoCredential.createMongoCRCredential(credential.getUser(), configuration.getDbName(), credential.getPassword().toCharArray())); } } MongoClientOptions.Builder optionsBuilder = new MongoClientOptions.Builder(); if (configuration.getConnectionsPerHost() != null) { optionsBuilder.connectionsPerHost(configuration.getConnectionsPerHost()); } if (configuration.getMaxWaitTime() != null) { optionsBuilder.maxWaitTime(configuration.getMaxWaitTime()); } if (configuration.getConnectionTimeout() != null) { optionsBuilder.connectTimeout(configuration.getConnectionTimeout()); } if (configuration.getSocketTimeout() != null) { optionsBuilder.socketTimeout(configuration.getSocketTimeout()); } if (configuration.getSocketKeepalive() != null) { optionsBuilder.socketKeepAlive(configuration.getSocketKeepalive()); } MongoClientOptions options = optionsBuilder.build(); mongoClient = new MongoClient(seeds, credentials, options); MongoDbFactory dbFactory = new SimpleMongoDbFactory(mongoClient, configuration.getDbName()); MappingMongoConverter converter = new MappingMongoConverter(dbFactory, new MongoMappingContext()); converter.setTypeMapper(new DefaultMongoTypeMapper(null)); mongoTemplate = new MongoTemplate(dbFactory, converter); mongoTemplate.setWriteResultChecking(WriteResultChecking.EXCEPTION); }
Project: allegro-intellij-templates File: $ Spring Data MongoDB Setup.java View source code | 6 votes |
@Bean(name = "mongoTemplate") public MongoTemplate createMongoTemplate() throws UnknownHostException { MongoClient mongoClient = new MongoClient(host, port); //TODO Configure additional MongoDB mongoClient settings if needed MongoDbFactory factory = new SimpleMongoDbFactory(mongoClient, database, new UserCredentials(username, password)); MappingMongoConverter converter = new MappingMongoConverter(new DefaultDbRefResolver(factory), new MongoMappingContext()); converter.setTypeMapper(new DefaultMongoTypeMapper(null)); return new MongoTemplate(factory, converter); }
Project: spring-data-mongodb File: SpringDataMongodbSerializerUnitTests.java View source code | 6 votes |
@Before public void setUp() { MongoMappingContext context = new MongoMappingContext(); this.converter = new MappingMongoConverter(dbFactory, context); this.serializer = new SpringDataMongodbSerializer(converter); }
Project: spring-data-mongodb File: PartTreeMongoQueryUnitTests.java View source code | 6 votes |
@Before @SuppressWarnings({ "unchecked", "rawtypes" }) public void setUp() { when(metadataMock.getDomainType()).thenReturn((Class) Person.class); when(metadataMock.getReturnedDomainClass(Matchers.any(Method.class))).thenReturn((Class) Person.class); mappingContext = new MongoMappingContext(); DbRefResolver dbRefResolver = new DefaultDbRefResolver(mock(MongoDbFactory.class)); MongoConverter converter = new MappingMongoConverter(dbRefResolver, mappingContext); when(mongoOperationsMock.getConverter()).thenReturn(converter); }
Project: spring-data-mongodb File: StringBasedMongoQueryUnitTests.java View source code | 6 votes |
@Before public void setUp() { when(operations.getConverter()).thenReturn(converter); this.converter = new MappingMongoConverter(factory, new MongoMappingContext()); }
Project: spring-data-mongodb File: ConvertingParameterAccessorUnitTests.java View source code | 6 votes |
@Before public void setUp() { this.context = new MongoMappingContext(); this.resolver = new DefaultDbRefResolver(factory); this.converter = new MappingMongoConverter(resolver, context); }
Project: members_cuacfm File: MongoConfig.java View source code | 6 votes |
@Bean public MappingMongoConverter mongoConverter() throws UnknownHostException { @SuppressWarnings("deprecation") MappingMongoConverter converter = new MappingMongoConverter(mongoDbFactory(), mongoMappingContext()); converter.setTypeMapper(mongoTypeMapper()); return converter; }
source - http://www.programcreek.com/java-api-examples/index.php?api=org.springframework.data.mongodb.core.convert.MappingMongoConverter
31 JULY 2011
Getting started with MongoDB and Spring Data
A LITTLE BACKGROUND INFORMATION
Most of you have probably have heard the term NoSQL before. The term is used in situations where you do not have a traditional relation database for storing information. There are many different sorts of NoSQL databases. To make a small summary these are probably the most well-known:
- Wide Column Stores: Hadoop and Cassandra
- Document Stores: CouchDB and MongoDB
- Key Value Store: Redis
- Eventually Consistent Key Value Store: Voldemort
- Graph Databases: Neo4J
The above types cover most of the differences, but for each type there are a lot of different implementations. For a better overview you might want to take a look at the NOSQL database website.
For my own experiment I chose to use MongoDB, since I had read a lot about it and it seemed quite easy to get started with.
MongoDB is as they describe it on their website:
A scalable, high-performance, open source, document-oriented database.The document-oriented aspect was one of the reasons why I chose MongoDB to start with. It allows you to store rich content with data structures inside your datastore.
GETTING STARTED WITH MONGODB
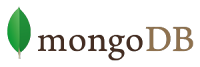
To begin with, I looked at the Quick start page for Mac OS X and I recommend you to do that too (unless you use a different OS). It will get you going and within a couple of minutes you'll have MongoDB up and running on your local machine.
MongoDB stores it's data by default in a certain location. Of course you can configure that, so I started MongoDB with the --dbpath parameter. This parameter will allow you to specificy your own storage location. It will look something like this:
$ ./mongodb-xxxxxxx/bin/mongod --dbpath=/Users/jreijn/Development/temp/mongodb/
If you do that you eventually will get a message saying:
Mon Jul 18 22:19:58 [initandlisten] waiting for connections on port 27017
Mon Jul 18 22:19:58 [websvr] web admin interface listening on port 28017
At this point MongoDB is running and we can proceed to the next step: using Spring Data to interact with MongoDB.
GETTING STARTED WITH SPRING DATA
The primary goal of the Spring Data project is to make it easier for developers to work with (No)SQL databases. The Spring Data project already has support for a number of the above mentioned NoSQL type of databases.Since we're now using MongoDB, there is a specific sub project that handles MongoDB interaction. To be able to use this in our project we first need to add a Maven dependency to our pom.xml.
1 2 3 4 5 | < dependency > < groupId >org.springframework.data</ groupId > < artifactId >spring-data-mongodb</ artifactId > < version >${spring.data.mongo.version}</ version > </ dependency > |
Looks easy right? Just one single Maven dependency. Of course in the end the spring-data-mongodb artifact depends on other artifacts which it will bring into your project. In this post I used version 1.0.2.RELEASE. Now on to some Java code!
For my first experiment I used a simple Person domain object that I'm going to query and persist inside the database. The Person class is quite simple and looks as follows.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | package com.jeroenreijn.mongodb.example.domain; import org.springframework.data.annotation.Id; import org.springframework.data.mongodb.core.mapping.Document; /** * A simple POJO representing a Person * */ @Document public class Person { @Id private String personId; private String name; private String homeTown; private int age; public Person(String name, int age) { this .name = name; this .age = age; } public String getPersonId() { return personId; } public void setPersonId( final String personId) { this .personId = personId; } public String getName() { return name; } public void setName( final String name) { this .name = name; } public int getAge() { return age; } public void setAge( final int age) { this .age = age; } public String getHomeTown() { return homeTown; } public void setHomeTown( final String homeTown) { this .homeTown = homeTown; } @Override public String toString() { return "Person [id=" + personId + ", name=" + name + ", age=" + age + ", home town=" + homeTown + "]" ; } } |
Now if you look at the class more closely you will see some Spring Data specific annotations like @Id and@Document . The @Document annotation identifies a domain object that is going to be persisted to MongoDB. Now that we have a persistable domain object we can move on to the real interaction.
For easy connectivity with MongoDB we can make use of Spring Data's MongoTemplate class. Here is a simple PersonRepository object that handles all 'Person' related interaction with MongoDB by means of the MongoTemplate.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | package com.jeroenreijn.mongodb.example; import java.util.Iterator; import java.util.List; import com.jeroenreijn.mongodb.example.domain.Person; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.stereotype.Repository; /** * Repository for {@link Person}s * */ @Repository public class PersonRepository { static final Logger logger = LoggerFactory.getLogger(PersonRepository. class ); @Autowired MongoTemplate mongoTemplate; public void logAllPersons() { List<Person> results = mongoTemplate.findAll(Person. class ); logger.info( "Total amount of persons: {}" , results.size()); logger.info( "Results: {}" , results); } public void insertPersonWithNameJohnAndRandomAge() { //get random age between 1 and 100 double age = Math.ceil(Math.random() * 100 ); Person p = new Person( "John" , ( int ) age); mongoTemplate.insert(p); } /** * Create a {@link Person} collection if the collection does not already exists */ public void createPersonCollection() { if (!mongoTemplate.collectionExists(Person. class )) { mongoTemplate.createCollection(Person. class ); } } /** * Drops the {@link Person} collection if the collection does already exists */ public void dropPersonCollection() { if (mongoTemplate.collectionExists(Person. class )) { mongoTemplate.dropCollection(Person. class ); } } } |
If you look at the above code you will see the MongoTemplate in action. There is quite a long list of method calls which you can use for inserting, querying and so on. The MongoTemplate in this case is @Autowiredfrom the Spring configuration, so let's have a look at the configuration.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | <? xml version = "1.0" encoding = "UTF-8" ?> xsi:schemaLocation="http://www.springframework.org/schema/beans <!-- Activate annotation configured components --> < context:annotation-config /> <!-- Scan components for annotations within the configured package --> < context:component-scan base-package = "com.jeroenreijn.mongodb.example" > < context:exclude-filter type = "annotation" expression = "org.springframework.context.annotation.Configuration" /> </ context:component-scan > <!-- Define the MongoTemplate which handles connectivity with MongoDB --> < bean id = "mongoTemplate" class = "org.springframework.data.mongodb.core.MongoTemplate" > < constructor-arg name = "mongo" ref = "mongo" /> < constructor-arg name = "databaseName" value = "demo" /> </ bean > <!-- Factory bean that creates the Mongo instance --> < bean id = "mongo" class = "org.springframework.data.mongodb.core.MongoFactoryBean" > < property name = "host" value = "localhost" /> </ bean > <!-- Use this post processor to translate any MongoExceptions thrown in @Repository annotated classes --> < bean class = "org.springframework.dao.annotation.PersistenceExceptionTranslationPostProcessor" /> </ beans > |
The MongoTemplate is configured with a reference to a MongoDBFactoryBean (which handles the actual database connectivity) and is setup with a database name used for this example.
Now that we have all components in place, let's get something in and out of MongoDB.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | package com.jeroenreijn.mongodb.example; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.context.ConfigurableApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * Small MongoDB application that uses spring data to interact with MongoDB. * */ public class MongoDBApp { static final Logger logger = LoggerFactory.getLogger(MongoDBApp. class ); public static void main( String[] args ) { logger.info( "Bootstrapping MongoDemo application" ); ConfigurableApplicationContext context = new ClassPathXmlApplicationContext( "META-INF/spring/applicationContext.xml" ); PersonRepository personRepository = context.getBean(PersonRepository. class ); // cleanup person collection before insertion personRepository.dropPersonCollection(); //create person collection personRepository.createPersonCollection(); for ( int i= 0 ; i< 20 ; i++) { personRepository.insertPersonWithNameJohnAndRandomAge(); } personRepository.logAllPersons(); logger.info( "Finished MongoDemo application" ); } } |
CONCLUSION
As you can see with Spring Data it's quite easy to get some basic functionality within only a couple of minutes. All the sources mentioned above and a working project can be found on GitHub. It was a fun first experiment and I already started working on a bit more advanced project, which combines Spring Data, MongoDB, HTML5 and CSS3. It will be on GitHub shortly together with another blog post here so be sure to come back.출처 - http://blog.jeroenreijn.com/2011/07/getting-started-with-mongodb-and-spring.html
Recently i seen no sql database . I was thought it’s some new concept until i read the documentation .
When i read the documentation i found that its similar to lotus notes documents concept , But more advance for scalability or performance .
Basic concept is .
- A Database have have multiple collections .
- Collection have multiple Documents.
- Documents can have embedded documents.
- documents have multiple fields.
- Each document have unique id called _id field.
Difference between lotus notes is , louts notes don’t have multiple collections , not able to embedded document into documents .
I hope that is enough as beginning .
For installng mongo db in ubuntu iam using
1 | sudo apt-get install mongodb |
then start the service using
1 | sudo mongod start |
then again
1 | mongo |
I am using netbeans to create new Maven web project with following pom files.
Basic idea about project iam storing Employee data in Mongo DB and check in shell that data is available.
I use maven for dependency and building the war file and deploy into netbeans buil in tomcat 7.
Following the pom files for dependency .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 | <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>ed.ws</groupId> <artifactId>mongospring</artifactId> <packaging>war</packaging> <version>1.0-SNAPSHOT</version> <name>mongospring</name> <dependencies> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> <scope>provided</scope> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.1</version> <scope>provided</scope> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.8.2</version> <scope>test</scope> </dependency> <!-- Spring framework --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>3.0.5.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>3.0.5.RELEASE</version> </dependency> <!-- mongodb java driver --> <dependency> <groupId>org.mongodb</groupId> <artifactId>mongo-java-driver</artifactId> <version>2.5.2</version> </dependency> <dependency> <groupId>org.springframework.data</groupId> <artifactId>spring-data-mongodb</artifactId> <version>1.0.0.M4</version> </dependency> <dependency> <groupId>cglib</groupId> <artifactId>cglib</artifactId> <version>2.2</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.0.2</version> <configuration> <source>1.5</source> <target>1.5</target> </configuration> </plugin> </plugins> </build> <repositories> <repository> <id>spring-snapshot</id> <name>Spring Maven SNAPSHOT Repository</name> </repository> <repository> <id>spring-milestone</id> <name>Spring Maven MILESTONE Repository</name> </repository> </repositories> </project> |
Next Step iam going to setup spring MVC and Applications Context .
Application context iam using beans.xml .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mongo="http://www.springframework.org/schema/data/mongo" xsi:schemaLocation="http://www.springframework.org/schema/context <!-- Scan components for annotations within the configured package --> <context:component-scan base-package="edu.samples.repository"> <context:exclude-filter type="annotation" expression="org.springframework.context.annotation.Configuration"/> </context:component-scan> <context:annotation-config/> <mongo:mongo host="127.0.1.1" port="27017"/> <mongo:db-factory id="mongoDbFactory" dbname="demo" mongo-ref="mongo" /> <bean id="template"> <constructor-arg name="mongoDbFactory" ref="mongoDbFactory"/> </bean> <bean/> </beans> |
Iam creating mongo db template in application context and annotate so it will auto inject into Contact Repository .
Iam creating Contact Repository to save Contact Object into Mongo Table.
Before insert into database , iam checking that collection available for entity name otherwise iam creating it .
Its not necessary that each entity type has one collection . One collection have multiple type of entity .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | /* * To change this template, choose Tools | Templates * and open the template in the editor. */ package edu.samples.repository; import edu.samples.mvc.basic.Contact; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.stereotype.Repository; /** * * @author home */ @Repository public class ContactRepository { @Autowired private MongoTemplate mongoTemplate; public void insertContact(Contact contact) { createPersonCollection(); //mongoTemplate.save(contact,"collections"); mongoTemplate.insert(contact); } public void createPersonCollection() { if (!mongoTemplate.collectionExists(Contact.class)) { mongoTemplate.createCollection(Contact.class); } } } |
Two way i can insert either use save or insert .
Save we can use collection name as parameter . Insert its use Entity class name for insert .
Following are my pojo for contact. @Document is base anotation. and @Id is id field .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | /* * To change this template, choose Tools | Templates * and open the template in the editor. */ package edu.samples.mvc.basic; import org.springframework.data.annotation.Id; import org.springframework.data.mongodb.core.mapping.Document; /** * * @author home */ @Document public class Contact { private String name; private String phone; private String email; private String department; private String designation; @Id private String id; public String getId() { return id; } public void setId(String id) { this.id = id; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public String getDesignation() { return designation; } public void setDesignation(String designation) { this.designation = designation; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } } |
Moving forward the setup for mvc .
Setting up common servlet as follows .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <!-- Scans the classpath of this application for @Components to deploy as beans --> <context:component-scan base-package="edu.samples.mvc.basic" /> <!-- Configures the @Controller programming model --> <mvc:annotation-driven /> <!-- Forwards requests to the "/" resource to the "welcome" view --> <mvc:view-controller path="/" view-name="welcome"/> <!-- Configures Handler Interceptors --> <mvc:interceptors> <!-- Changes the locale when a 'locale' request parameter is sent; e.g. /?locale=de --> <bean /> </mvc:interceptors> <!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources/ directory --> <mvc:resources mapping="/resources/**" location="/resources/" /> <!-- Saves a locale change using a cookie --> <bean id="localeResolver" /> <!-- Application Message Bundle --> <bean id="messageSource"> <property name="basename" value="/WEB-INF/messages/messages" /> <property name="cacheSeconds" value="0" /> </bean> <!-- Resolves view names to protected .jsp resources within the /WEB-INF/views directory --> <bean> <property name="prefix" value="/WEB-INF/"/> <property name="suffix" value=".jsp"/> </bean> </beans> |
Iam using resources to keep my commong css and js files .
All other settings are commong for Spring MVC 3 setups. Nothing fancy .
next is controller code.
I am using /basic as url , to display and update contact details .
When post the /basic controller from front end , the contact Pojo already updated with value .
When calling insertContact method , it will insert the data into mongo db.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | /* * To change this template, choose Tools | Templates * and open the template in the editor. */ package edu.samples.mvc.basic; import edu.samples.repository.ContactRepository; import org.apache.log4j.Logger; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.validation.BindingResult; import org.springframework.validation.ObjectError; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; /** * * @author home */ @Controller @RequestMapping(value = "/basic") public class BasicController { @Autowired ContactRepository repository; private static final Logger logger = Logger.getLogger(BasicController.class); @RequestMapping(method = RequestMethod.GET) public String getCreateForm(Model model) { logger.info("started basecontroller"); logger.debug("base controller debug"); model.addAttribute("testing"); model.addAttribute("contactbean", new Contact()); return "basic/basicForm"; } @RequestMapping(method = RequestMethod.POST) public String createContact(@ModelAttribute("contactbean")Contact contact, BindingResult result) { System.out.println("started uploading" + contact.getName()); if (result.hasErrors()) { for (ObjectError error : result.getAllErrors()) { System.err.println("Error in uploading: " + error.getCode() + " - " + error.getDefaultMessage()); } } repository.insertContact(contact); return "basic/basicForm"; } } |
I am using Contact pojo as front end pojo and back end pojo for inserting back to database .
Final one jsp page code .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 | <%-- Document : basicForm Created on : Oct 11, 2011, 9:03:28 PM Author : home --%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <%@ taglib uri="http://www.springframework.org/tags" prefix="s" %> <%@ taglib uri="http://www.springframework.org/tags/form" prefix="form" %> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>JSP Page</title> <title>spring-mvc-showcase</title> <link href="<c:url value="/resources/css/custom-theme/jquery-ui-1.8.16.custom.css" />" rel="stylesheet" type="text/css" /> <script type="text/javascript" src="<c:url value="/resources/js/jquery-1.6.2.min.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jquery-ui-1.8.16.custom.min.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jquery.ui.button.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jquery.ui.core.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jquery.ui.widget.js" />"></script> <script> $(document).ready(function() { $("button").button(); }); </script> <style type="text/css"> *{ margin:0; padding:0; font:bold 12px "Lucida Grande", Arial, sans-serif; } body { padding: 10px; } #status{ width:250px; padding:10px; outline:none; height:36px; } </style> </head> <body> <form:form modelAttribute="contactbean" method="post" > <h1>Its for saving Employee details back to MongoDB/h1> <table> <tr> <td> <div> Your name </div></td> <td> <form:input path="name"/> </br></td> </tr> <tr> <td> <div> Your email account </div></td> <td> <form:input path="email"/> </br></td> </tr> <tr> <td> <div> Your mobile phone </div></td> <td> <form:input path="phone"/> </td> </tr> <tr> <td><div> Your Department </div></td> <td> <form:input path="department"/> </td> </tr> <tr> <td><div> Designation </div></td> <td> <form:input path="designation"/> </td> </tr> </table> <button>Click here to save</button> </form:form> </button> </body> </html> |
Iam using jquery library . but i keep the library in the resources folder .
That functionality is new in spring MVC .
Now time to deploy the application in tomcat
Build the application and deploy in the tomcat using netbeans build it tomcat 7.
The front page looks like as follows and click on green button to save the data .
Once save the data , iam looking on shell to verify the data.
As we are create collection based on entity type , the collection name should be contact.
1 2 3 4 5 | public void createPersonCollection() { if (!mongoTemplate.collectionExists(Contact.class)) { mongoTemplate.createCollection(Contact.class); } } |
Finally result showing in the terminal
출처 - http://sjohn4.wordpress.com/2011/10/20/no-sql-db-mongodb-introduction/
Spring Data MongoDB Hello World Example
In this tutorial, we show you how to use “SpringData for MongoDB” framework, to perform CRUD operations in MongoDB, via Spring’s annotation and XML schema.
Article is updated to use latest SpringData v 1.2.0.RELEASE, it was v1.0.0.M2.
Tools and technologies used :
- Spring Data MongoDB – 1.2.0.RELEASE
- Spring Core – 3.2.2.RELEASE
- Java Mongo Driver – 2.11.0
- Eclipse – 4.2
- JDK – 1.6
- Maven – 3.0.3
P.S Spring Data requires JDK 6.0 and above, and Spring Framework 3.0.x and above.
1. Project Structure
A classic Maven’s style Java project directory structure.
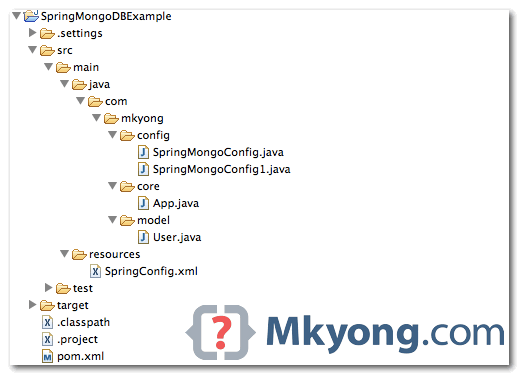
2. Dependency
The following libraries are required :
Currently, the “
spring-data-mongodb
” jar is only available in “http://maven.springframework.org/milestone“, so, you have to declare this repository also.Updated on 13/09/2012spring-data-mongodb
is available at the Maven central repository, Spring repository is no longer required.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong.core</groupId> <artifactId>SpringMongoDBExample</artifactId> <packaging>jar</packaging> <version>1.0</version> <name>SpringMongoExample</name> <url>http://maven.apache.org</url> <dependencies> <!-- Spring framework --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>3.2.2.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>3.2.2.RELEASE</version> </dependency> <!-- mongodb java driver --> <dependency> <groupId>org.mongodb</groupId> <artifactId>mongo-java-driver</artifactId> <version>2.11.0</version> </dependency> <!-- Spring data mongodb --> <dependency> <groupId>org.springframework.data</groupId> <artifactId>spring-data-mongodb</artifactId> <version>1.2.0.RELEASE</version> </dependency> <dependency> <groupId>cglib</groupId> <artifactId>cglib</artifactId> <version>2.2.2</version> </dependency> </dependencies> <build> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.0</version> <configuration> <source>1.6</source> <target>1.6</target> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-eclipse-plugin</artifactId> <version>2.9</version> <configuration> <downloadSources>true</downloadSources> <downloadJavadocs>true</downloadJavadocs> </configuration> </plugin> </plugins> </build> </project>
3. Spring Configuration, Annotation and XML
Here, we show you two ways to configure Spring data and connect to MongoDB, via annotation and XML schema.
Refer to this official reference Connecting to MongoDB with Spring.
3.1 Annotation
Extends the AbstractMongoConfiguration
is the fastest way, it helps to configure everything you need to start, likemongoTemplate
object.
package com.mkyong.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.mongodb.config.AbstractMongoConfiguration; import com.mongodb.Mongo; import com.mongodb.MongoClient; @Configuration public class SpringMongoConfig extends AbstractMongoConfiguration { @Override public String getDatabaseName() { return "yourdb"; } @Override @Bean public Mongo mongo() throws Exception { return new MongoClient("127.0.0.1"); } }
Alternatively, I prefer this one, more flexible to configure everything.
package com.mkyong.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.mongodb.MongoDbFactory; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.data.mongodb.core.SimpleMongoDbFactory; import com.mongodb.MongoClient; @Configuration public class SpringMongoConfig1 { public @Bean MongoDbFactory mongoDbFactory() throws Exception { return new SimpleMongoDbFactory(new MongoClient(), "yourdb"); } public @Bean MongoTemplate mongoTemplate() throws Exception { MongoTemplate mongoTemplate = new MongoTemplate(mongoDbFactory()); return mongoTemplate; } }
And load it with AnnotationConfigApplicationContext
:
ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringMongoConfig.class); MongoOperations mongoOperation = (MongoOperations)ctx.getBean("mongoTemplate");
3.2 XML Schema
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mongo="http://www.springframework.org/schema/data/mongo" xsi:schemaLocation="http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/data/mongo http://www.springframework.org/schema/data/mongo/spring-mongo-1.0.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <mongo:mongo host="127.0.0.1" port="27017" /> <mongo:db-factory dbname="yourdb" /> <bean id="mongoTemplate" class="org.springframework.data.mongodb.core.MongoTemplate"> <constructor-arg name="mongoDbFactory" ref="mongoDbFactory" /> </bean> </beans>
And include it with Spring’s GenericXmlApplicationContext
:
ApplicationContext ctx = new GenericXmlApplicationContext("SpringConfig.xml"); MongoOperations mongoOperation = (MongoOperations)ctx.getBean("mongoTemplate");
Actually, both are doing the same thing, it’s just based on personal preferences. Personally, I like XML to configure things.
4. User Model
An User object, annotated @Document – which collection to save. Later, we show you how to use Spring data to bind this object to / from MongoDB.
package com.mkyong.model; import org.springframework.data.annotation.Id; import org.springframework.data.mongodb.core.mapping.Document; @Document(collection = "users") public class User { @Id private String id; String username; String password; //getter, setter, toString, Constructors
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public User(String username, String password) {
super();
this.username = username;
this.password = password;
}
@Override
public String toString() {
return "User [id=" + id + ", username=" + username + ", password=" + password + "]";
}
}
5. Demo – CRUD Operations
Full example to show you how to use Spring data to perform CRUD operations in MongoDB. The Spring data APIs are quite clean and should be self-explanatory.
package com.mkyong.core; import java.util.List; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.data.mongodb.core.MongoOperations; import org.springframework.data.mongodb.core.query.Criteria; import org.springframework.data.mongodb.core.query.Query; import org.springframework.data.mongodb.core.query.Update; import com.mkyong.config.SpringMongoConfig; import com.mkyong.model.User; //import org.springframework.context.support.GenericXmlApplicationContext; public class App { public static void main(String[] args) { // For XML //ApplicationContext ctx = new GenericXmlApplicationContext("SpringConfig.xml"); // For Annotation ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringMongoConfig.class); MongoOperations mongoOperation = (MongoOperations) ctx.getBean("mongoTemplate"); User user = new User("mkyong", "password123"); // save mongoOperation.save(user); // now user object got the created id. System.out.println("1. user : " + user); // query to search user Query searchUserQuery = new Query(Criteria.where("username").is("mkyong")); // find the saved user again. User savedUser = mongoOperation.findOne(searchUserQuery, User.class); System.out.println("2. find - savedUser : " + savedUser); // update password mongoOperation.updateFirst(searchUserQuery, Update.update("password", "new password"),User.class); // find the updated user object User updatedUser = mongoOperation.findOne(searchUserQuery, User.class); System.out.println("3. updatedUser : " + updatedUser); // delete mongoOperation.remove(searchUserQuery, User.class); // List, it should be empty now. List<User> listUser = mongoOperation.findAll(User.class); System.out.println("4. Number of user = " + listUser.size()); } }
Output
1. user : User [id=516627653004953049d9ddf0, username=mkyong, password=password123] 2. find - savedUser : User [id=516627653004953049d9ddf0, username=mkyong, password=password123] 3. updatedUser : User [id=516627653004953049d9ddf0, username=mkyong, password=new password] 4. Number of user = 0
Download Source Code
References
- Spring data for MongoDB
- Connecting to MongoDB with Spring
- Oreilly Spring data mongodb tutorial
- Another good Spring data mongodb tutorial
출처 - http://www.mkyong.com/mongodb/spring-data-mongodb-hello-world-example/
Friday, June 29, 2012
Spring Data MongoDB Example
1. Setting up Spring Mongo
The Spring API provides an abstract Spring Java Config class, org.springframework.data.mongodb.config.AbstractMongoConfiguration. This class requires the following methods to be implemented, getDatabaseName() and mongo() which returns a Mongo instance. The class also has a method to create a MongoTemplate. Extending the mentioned class, the following is a Mongo Config:
01.
@Configuration
02.
@PropertySource
(
"classpath:/mongo.properties"
)
03.
public
class
MongoConfig
extends
AbstractMongoConfiguration {
04.
05.
private
Environment env;
06.
07.
@Autowired
08.
public
void
setEnvironment(Environment environment) {
09.
this
.env = environment;
10.
}
11.
12.
@Bean
13.
public
Mongo mongo()
throws
UnknownHostException, MongoException {
14.
// create a new Mongo instance
15.
return
new
Mongo(env.getProperty(
"host"
));
16.
}
17.
18.
@Override
19.
public
String getDatabaseName() {
20.
return
env.getProperty(
"databaseName"
);
21.
}
22.
}
2. Model Objects and Annotations
As per my former example, we have four primary objects that comprise our domain. A Product in the system such as an XBOX, WII, PS3 etc. ACustomer who purchases items by creating an Order. An Order has references to LineItem(s) which in turn have a quantity and a reference to a Product for that line.
2.1 The Order model object looks like the following:
01.
// @Document to indicate the orders collection
02.
@Document
(collection =
"orders"
)
03.
public
class
Order {
04.
// Identifier
05.
@Id
06.
private
ObjectId id;
07.
08.
// DB Reference to a Customer. This is a Link to a Customer from the Customer collection
09.
@DBRef
10.
private
Customer customer;
11.
12.
// Line items are part of the Order and do not exist independently of the order
13.
private
List<LineItem> lines;
14.
...
15.
}
3. Implementing the DAO pattern
One can use the Mongo Template directly or extend or compose a DAO class that provides standard CRUD operations. I have chosen the extension route for this example. The Spring Mongo API provides an interface org.springframework.data.repository.CrudRepository that defines methods as indicated by the name for CRUD operations. An extention to this interface is theorg.springframework.data.repository.PagingAndSortingRepository which provides methods for paginated access to the data. One implementation of these interfaces is the SimpleMongoRepository which the DAO implementations in this example extend:
01.
// OrderDao interface exposing only certain operations via the API
02.
public
interface
OrderDao {
03.
Order save(Order order);
04.
05.
Order find(ObjectId orderId);
06.
07.
List<Order> findOrdersByCustomer(Customer customer);
08.
09.
List<Order> findOrdersWithProduct(Product product);
10.
}
11.
12.
public
class
OrderDaoImpl
extends
SimpleMongoRepository<Order, ObjectId>
implements
OrderDao {
13.
14.
public
OrderDaoImpl(MongoRepositoryFactory factory, MongoTemplate template) {
15.
super
(
new
MongoRepositoryFactory(template).<Order, ObjectId>getEntityInformation(Order.
class
), template);
16.
}
17.
18.
@Override
19.
public
List<Order> findOrdersByCustomer(Customer customer) {
20.
// Create a Query and execute the same
21.
Query query = Query.query(Criteria.where(
"customer"
).is(customer));
22.
23.
// Note the equivalent of Hibernate where one would do getHibernateTemplate()...
24.
return
getMongoOperations().find(query, Order.
class
);
25.
}
26.
27.
@Override
28.
public
List<Order> findOrdersWithProduct(Product product) {
29.
// Where the lines matches the provided product
30.
Query query = Query.query(Criteria.where(
"lines.product.$id"
).is(product));
31.
return
getMongoOperations().find(query, Order.
class
);
32.
}
33.
}
1.
//1.0.2.RELEASE
2.
public
<T> T save(T entity) {
3.
}
4.
5.
// 1.1.0.M1
6.
public
<S
extends
T> S save(S entity) {
7.
}
1.
@Override
2.
@SuppressWarnings
(
"unchecked"
)
3.
public
Order save(Order order) {
4.
return
super
.save(order);
5.
}
4. Configuration for the DAO's
A Java Config is set up that wires up the DAO's
01.
@Configuration
02.
@Import
(MongoConfig.
class
)
03.
public
class
DaoConfig {
04.
@Autowired
05.
private
MongoConfig mongoConfig;
06.
07.
@Bean
08.
public
MongoRepositoryFactory getMongoRepositoryFactory() {
09.
try
{
10.
return
new
MongoRepositoryFactory(mongoConfig.mongoTemplate());
11.
}
12.
catch
(Exception e) {
13.
throw
new
RuntimeException(
"error creating mongo repository factory"
, e);
14.
}
15.
}
16.
17.
@Bean
18.
public
OrderDao getOrderDao() {
19.
try
{
20.
return
new
OrderDaoImpl(getMongoRepositoryFactory(), mongoConfig.mongoTemplate());
21.
}
22.
catch
(Exception e) {
23.
throw
new
RuntimeException(
"error creating OrderDao"
, e);
24.
}
25.
}
26.
...
27.
}
5. Life Cycle Event Listening
The Order object has the following two properties, createDate and lastUpdate date which are updated prior to persisting the object. To listen for life cycle events, an implemenation of the org.springframework.data.mongodb.core.mapping.event.AbstractMongoEventListener can be provided that defines methods for life cycle listening. In the example provide we override the onBeforeConvert() method to set the create and lastUpdateDate properties.
01.
public
class
OrderSaveListener
extends
AbstractMongoEventListener<Order> {
02.
/**
03.
* This method is responsible for any code before updating to the database object.
04.
*/
05.
@Override
06.
public
void
onBeforeConvert(Order order) {
07.
order.setCreationDate(order.getCreationDate() ==
null
?
new
Date() : order.getCreationDate());
08.
order.setLastUpdateDate(order.getLastUpdateDate() ==
null
? order.getCreationDate() :
new
Date());
09.
}
10.
}
6. Indexing
The Spring Data API for Mongo has support for Indexing and ensuring the presence of indices as well. An index can be created using the MongoTemplate via:
1.
mongoTemplate.ensureIndex(
new
Index().on(
"lastName"
,Order.ASCENDING), Customer.
class
);
7. JPA Cross Domain or Polyglot Persistence
If you wish to re-use your JPA objects to persist to Mongo, then take a look at the following article for further information about the same.
http://www.littlelostmanuals.com/2011/10/example-cross-store-with-mongodb-and.html
8. Map Reduce
{ "_id" : ObjectId("4e5ff893c0277826074ec533"), "commenterId" : "jamesbond", "comment":"James Bond lives in a cave", "country" : "INDIA"] } { "_id" : ObjectId("4e5ff893c0277826074ec535"), "commenterId" : "nemesis", "comment":"Bond uses Walther PPK", "country" : "RUSSIA"] } { "_id" : ObjectId("4e2ff893c0277826074ec534"), "commenterId" : "ninja", "comment":"Roger Rabit wanted to be on Geico", "country" : "RUSSIA"] }The map reduce works of JSON files for the mapping and reducing functions. For the mapping function we have mapComments.js which only maps certain words:
function () { var searchingFor = new Array("james", "2012", "cave", "walther", "bond"); var commentSplit = this.comment.split(" "); for (var i = 0; i < commentSplit.length; i++) { for (var j = 0; j < searchingFor.length; j++) { if (commentSplit[i].toLowerCase() == searchingFor[j]) { emit(commentSplit[i], 1); } } } }For the reduce operation, another javascript file reduce.js:
function (key, values) { var sum = 0; for (var i = 0; i < values.length; i++) { sum += values[i]; } return sum; }The mapComment.js and the reduce.js are made available in the classpath and the M/R operation is invoked as shown below:
1.
public
List<ValueObject> mapReduce() {
2.
MapReduceResults<ValueObject> results = getMongoOperations().mapReduce(
"comments"
,
"classpath:mapComment.js"
,
"classpath:reduce.js"
, ValueObject.
class
);
3.
4.
return
Lists.<ValueObject>newArrayList(results);
5.
}
ValueObject [id=2012, value=119.0] ValueObject [id=Bond, value=258.0] ValueObject [id=James, value=241.0] ValueObject [id=Walther, value=134.0] ValueObject [id=bond, value=117.0] ValueObject [id=cave, value=381.0]
Conclusion
Example
Enjoy!
출처 - http://sleeplessinslc.blogspot.kr/2012/06/spring-data-mongodb-example.html
Pre requests:
- eclipse and maven plugin for eclipse
- maven ( command line, optional )
- mongodb
- java jdk 1.6
In eclipse create a new maven project
- File - New - Others - Maven - Maven Project
- Check create a simple project
- select .war and fill the blanks
At the end of this process you should have a java project and minimal pom.xml
Add the spring, springdata and mongodb dependencies in pom.xml
Create controler, service, model and repository packages.
Model package will include simple POJO classes. These classes will be modeled over the mongoDB collections.
User.java Class: package com.flgor.model; import org.springframework.data.annotation.Id; public class User { @Id String id; String name; String password; public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
Repository package will contain only interfaces. These interfaces will extend MongoRepository interface.
Into these interfaces we can declare Query methods. ( check springdata documentation for details )
This is an exemple:
IUserRepository.java class: package com.flgor.repository; import java.util.List; import org.springframework.data.document.mongodb.repository.MongoRepository; import com.flgor.model.User; public interface IUserRepository extends MongoRepository< User, String > { List< User > findByName(String user); }
In the next file are created the mongoTemplate and the mongorepositories beans.
mongo-config.xml < ?xml version="1.0" encoding="UTF-8"? > < beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:mongo="http://www.springframework.org/schema/data/mongo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/data/mongo http://www.springframework.org/schema/data/mongo/spring-mongo-1.0.xsd" > < mongo:repositories base-package="com.flgor.repository" / > < mongo:mongo host="localhost" port="27017" / > < bean id="mongoTemplate" class="org.springframework.data.document.mongodb.MongoTemplate" > < constructor-arg ref="mongo" / > < constructor-arg name="databaseName" value="tutorialDB" / > < constructor-arg name="defaultCollectionName" value="user" / > < /bean > < /beans >
Using spring data ( mongotemplate and mongorepository ) it's very eazy and natural to work over the mongo database.
Next is a simple service.java exemple (service package ). It's implemented mongo collection clean, insert, findall and find by name inside the initialise method.
package com.flgor.service; import java.util.List; import javax.annotation.PostConstruct; import org.apache.log4j.Logger; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.document.mongodb.MongoTemplate; import org.springframework.stereotype.Repository; import org.springframework.transaction.annotation.Transactional; import com.flgor.model.User; import com.flgor.repository.IProductRepository; import com.flgor.repository.IUserRepository; import com.flgor.model.Product; @Repository @Transactional public class ApplicationService { @Autowired private IProductRepository productRepository; @Autowired private IUserRepository userRepository; @Autowired private MongoTemplate mongoTemplate; private final static Logger logger = Logger .getLogger(ApplicationService.class); @PostConstruct public void initialise() { // Clean User and Product Database mongoTemplate.dropCollection("user"); productRepository.deleteAll(); // Add an user using MongoRepository User user = new User(); user.setName("admin"); user.setPassword("admin"); userRepository.save(user); // Add products using MongoTemplate for (int i = 0; i < 10; i++) { Product product = new Product(); product.setName("product " + i); product.setPrice((float) (i * 100)); mongoTemplate.save("product", product); } List < Product > productList = productRepository.findAll(); for (Product prod : productList) { logger.info(prod.getName()); } // findByName is only declared in IUserRepository // the magic is done automatically by Spring List < User > userList = userRepository.findByName("admin"); logger.info("First user is: " + userList.get(0).getName()); } }
For moment controller it's not implemented. Also there is no viewer resolver.
Start mongodb (./bin/mongod )
Start the tutorial with mvn tomcat:run
mvn clean install may help.
The applicationService output at startup:
1423 [main] INFO com.flgor.service.ApplicationService - product 0 1423 [main] INFO com.flgor.service.ApplicationService - product 1 1423 [main] INFO com.flgor.service.ApplicationService - product 2 1423 [main] INFO com.flgor.service.ApplicationService - product 3 1423 [main] INFO com.flgor.service.ApplicationService - product 4 1423 [main] INFO com.flgor.service.ApplicationService - product 5 1423 [main] INFO com.flgor.service.ApplicationService - product 6 1423 [main] INFO com.flgor.service.ApplicationService - product 7 1423 [main] INFO com.flgor.service.ApplicationService - product 8 1423 [main] INFO com.flgor.service.ApplicationService - product 9 1440 [main] INFO com.flgor.service.ApplicationService - First user is: admin
Into the the mongo database will be crated a user collection( with 1 entry ) and product collection( with 10 entries).
A tipical servletName-servlet.xml for SPRING MVC project:
< ?xml version="1.0" encoding="UTF-8"? > < beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd" > < import resource="classpath:tutorialApplicationContext.xml" / > < context:annotation-config / > < context:component-scan base-package="com.flgor" / > < mvc:annotation-driven / > < /beans >
This is how the pom.xml should look like.
< project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd" > < modelVersion > 4.0.0 < /modelVersion > < groupId > com.flgor < /groupId > < artifactId > tutorial < /artifactId > < version > 0.0.1 < /version > < packaging > war < /packaging > < name > mongotutorial < /name > < description > spring data tutorial < /description > < dependencies > < dependency > < groupId > javax.annotation < /groupId > < artifactId > jsr250-api < /artifactId > < version > 1.0 < /version > < /dependency > < dependency > < groupId > jstl < /groupId > < artifactId > jstl < /artifactId > < version > 1.1.2 < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > taglibs < /groupId > < artifactId > standard < /artifactId > < version > 1.1.2 < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > junit < /groupId > < artifactId > junit < /artifactId > < version > 4.8.1 < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > log4j < /groupId > < artifactId > log4j < /artifactId > < version > 1.2.14 < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > org.springframework < /groupId > < artifactId > spring-web < /artifactId > < version > 3.0.5.RELEASE < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > org.springframework < /groupId > < artifactId > spring-core < /artifactId > < version > 3.0.5.RELEASE < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > org.springframework < /groupId > < artifactId > spring-tx < /artifactId > < version > 3.0.5.RELEASE < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > org.springframework < /groupId > < artifactId > spring-webmvc < /artifactId > < version > 3.0.5.RELEASE < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > org.springframework < /groupId > < artifactId > spring-aop < /artifactId > < version > 3.0.5.RELEASE < /version > < type > jar < /type > < scope > compile < /scope > < /dependency > < dependency > < groupId > org.springframework.data < /groupId > < artifactId > spring-data-mongodb < /artifactId > < version > 1.0.0.M2 < /version > < /dependency > < /dependencies > < build > < finalName > spring-tutorial < /finalName > < plugins > < plugin > < groupId > org.apache.maven.plugins < /groupId > < artifactId > maven-compiler-plugin < /artifactId > < version > 2.3.2 < /version > < configuration > < source > 1.5 < /source > < target > 1.5 < /target > < /configuration > < /plugin > < /plugins > < /build > < repositories > < repository > < id > spring-maven-milestone < /id > < name > Springframework Maven Repository < /name > < url > http://maven.springframework.org/milestone < /url > < /repository > < /repositories > < /project >
The whole project can be downloaded from this location:
http://code.google.com/p/bet-alarm/downloads/list
Use Import -> Maven -> Existing Maven Project to import the project.
Used links:
http://static.springsource.org/spring-data/data-document/docs/1.0.0.M3/reference/html/
http://krams915.blogspot.com/2011/04/spring-data-mongodb-revision-for-100m2.html
출처 - http://flgor.blogspot.kr/2011/08/spring-3-mvc-with-mongo-db-using.html
Spring Data – Part 3: MongoDB
In this part of my blog series I’m going to show how easy it is to access a MongoDB datastore with Spring Data MongoDB.
MongoDB
MongoDB is a so called NoSQL datastore for document-oriented storage. A good place to start with MongoDB is the Developer Zone on the project’s homepage. After downloading and installing MongoDB we create a folder for data storage and start the server with
and are welcomed by a web admin interface at http://localhost:28017/. To play around with MongoDB, use the interactive mongo shell:
We display the names of the databases, than the collections (a collection is a logical namespace) inside the default databasetest
. After that, we persists three documents in JSON notation. Doing so we observe:
- each document has a unique id
- there may be more than one document holding the same attribute set in the same collection
- documents with different structures can be stored in the same collection
So a collection is really not the same thing as a table of a relational database. We also have no support for ACID transaction handling. Welcome to the cloud!
Spring Data MongoDB
Spring Data MongoDB works basically the same way as Spring Data JPA: you define your custom repository finders by writing only interface methods and Spring provides an implementation at runtime. The basic CRUD operation are supported without the need to write a single line of code.
Configuration
First of all we let Maven download the latest realeae version of Spring Data MongoDB:
Using the mongo
namespace your Spring application context can be configured quite easy:
The connection to our MongoDB server and the database to use are configured with the <mongo:db-factory .../>
tag. For fine tuning of the connection (connection pooling, clustering etc.) use the elements <mongo:mongo>
und<mongo:options/>
instead. Then we define a template that refers our DB factory. Finally we have to configure the package holding our repository interfaces (same as with Spring Data JPA). By default the only MongoDBTemplate inside the application context is used. If there are more than one template, you can specify which one to use with <mongo:repositories mongo-template-ref="...">
.
Example
Similar to the blog post on Spring Data JPA we like to persist some simple User
objects:
The annotations are not required. But to define an index we have to use the @Indexed
annotation. To begin with we use a very simple repository …
… to save our first documents:
We use the mongo shell to check if our documents were persisted:
You may have noticed that a collection named user
was created on the fly. If you want a non-default collection name (the lowercase name of the Java class), use the document annotation: @Document(collection="...")
. The full qualified class name is persisted with the _class
attribute. There are two indexes now: the default index for the id attribute and the index generated from the class attribute fullName
with the @Indexed
annotation.
Now we write some more custom finders:
With the @Query
annotation you can define random queries in MongoDB syntax. The second query shows a finder that provides a search with regular expressions. When writing your first queries the comparison between MongoDB and SQL can be very helpful.
The complete source code of the example can be downloaded from Github.
MongoDBTemplate
Not all MongoDB features are exposed with the interface based repository approach. If you want to manage collections or usemap/reduce, you have to use the API of the MongoDBTemplate
.
Summary
After a short introduction to MongoDB we were able to persist the first object very fast using Spring Data MongoDB. After that, we wrote custom finders with just a few lines of code.
A Spring application using Spring Data MongoDB as a persistence layer can be deployed to a cloud platform like CloudFoundry. This blog post show how easy that can be done.
What happened before?
Part 1: Spring Data Commons
Part 2: Spring Data JPA
What’s next?
Expect upcoming blog posts on Spring Data Neo4j and Spring GemFire.
출처 - http://blog.codecentric.de/en/2012/02/spring-data-mongodb/
출처 - http://yunsunghan.tistory.com/579
'Framework & Platform > Spring' 카테고리의 다른 글
spring data - Spring Data MongoDB Remove _class field (0) | 2013.06.03 |
---|---|
spring data - mongoTemplate CRUD example (0) | 2013.05.29 |
spring - ehcache config (0) | 2013.04.16 |
spring - PropertyPlaceholderConfigurer(Property 값 읽어오기) (0) | 2013.03.10 |
spring oxm (0) | 2013.01.10 |